Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial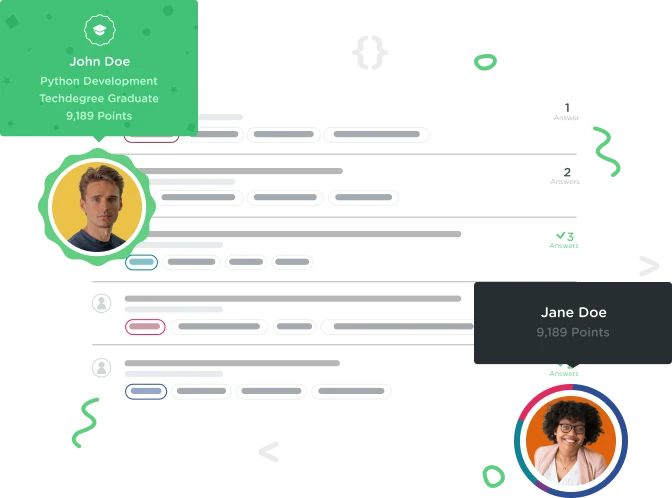

Jonathan Whelchel
2,096 PointsY or n not defined when trying to play game again
import random
secret_num = random.randint(1, 10)
guesses = []
def game():
while len(guesses) < 5:
try:
guess = int(input("Pick a number between 1 and 10: "))
except ValueError:
print("{} isn't a number!".format(guess))
else:
if guess == secret_num:
print("You got it! My number was {}".format(secret_num))
break
elif guess < secret_num:
print("My number is higher than {}".format(guess))
else:
print("My number is lower than {}".format(guess))
guesses.append(guess)
else:
print("You didnt'get it! My number was {}".format(secret_num))
play_again = input("Do you want to play again? Y/n ")
if play_again.lower() != 'n':
game()
else:
print("Bye!")
game()
[MOD: added ```python formatting -cf]
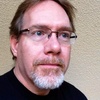
Chris Freeman
Treehouse Moderator 68,460 PointsIf you're running Python 3 ignore the previous comment.
4 Answers
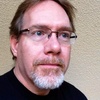
Chris Freeman
Treehouse Moderator 68,460 PointsThere are a few issues with the code:
- the list
guesses
does not get reset for new games. This leads to the eventual condition wherelen(guesses)
will not be less than 5. - the try/except code fails because even though the ValueError is caught,
guess
is still undefined and will raise a second error during the print statement
Post back if you need more help. Good luck!!!

Jonathan Whelchel
2,096 PointsI am running python 3. If I put the n with '' in my code why do I have to put them in when I answer the prompt.
Also, If I put in "Y" I don't get an error but the game doesn't start over.
Do you want to play again? Y/n "y" You didnt'get it! My number was 10

Jonathan Whelchel
2,096 PointsI am. But your answer still worked. Once I put ‘n’ it printed bye. So I don’t know why I have to put it in a string. However when I put in ‘y’ it prints the last line which is ‘you didn’t get it...’ and it asked me if I want to play again.

Jonathan Whelchel
2,096 PointsThanks for your help. So in this exercise is the game not meant to be reset?
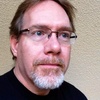
Chris Freeman
Treehouse Moderator 68,460 PointsReset the game by adding a guesses = []
before calling game()
again
Chris Freeman
Treehouse Moderator 68,460 PointsChris Freeman
Treehouse Moderator 68,460 PointsAre you running under Python 2? If so, you need to provide quoted strings as input: "n" and "y".