Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial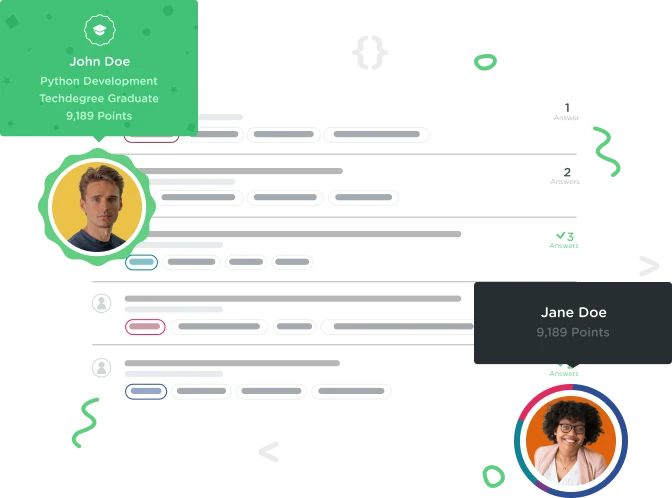
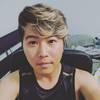
James Han
9,419 PointsTypecasting and instanceof, having trouble understanding even though I passed the quiz.
So, I passed the short quiz but I don't understand what's going on. I used the below code to get it to work, but don't understand it completely.
public static String getTitleFromObject(Object obj) {
// Fix this result variable to be the correct string.
String result;
if (obj instanceof String) {
result = obj.toString();
} else {
result = "";
}
//String result = "";
return result;
}
I assumed i could just do:
String result;
if (obj instanceof String) {
result = obj;
}
How I read it: if obj is an instance of String, set obj to result. What am I missing?
3 Answers
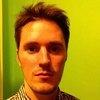
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsAs far as Java knows, obj
is of type Object
and result
, or the return type, is of type String
. Even though we're inside of block where we've explicitly checked the type of the object and confirmed that it is a String
, the compiler isn't smart enough to know that. So it yells at us that we're trying to assign an Object to a String. So we can cast with (String)
it to a String where we're basically saying at this point I've checked and I promise you that it will be a string. The compiler is trying to protect us from mistakes where we pass around the wrong type by accident, and usually it's very good at inferring what the type will be, but sometimes it's not smart enough and we need to provide it with information that we know because we know more than it.
if (obj instanceof String) {
return (String) obj;
} else {
return "";
}
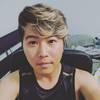
James Han
9,419 PointsI think i'm getting it a little by little. Could I have also done:
String result;
if (obj instanceof String) {
result = (String) obj;
}
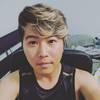
James Han
9,419 Points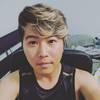
James Han
9,419 PointsGot it! Thanks!
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsBrendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsCan you link to the quiz?