Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial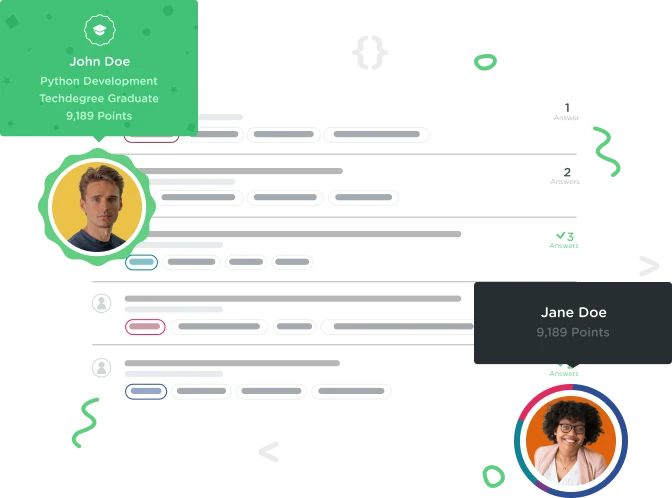
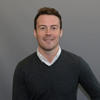
Lex Dunko
Data Analysis Techdegree Student 4,449 PointsTreeStory Extra Credit Contains not working
Ok, so this is driving me mental. I've looked around the community and online to see if I can find a solution - I can't.
So the code below works, if I type in a naughty word from my list below, it will reject it and ask for you to type it again. It also works regardless of the case. however, if I type in a longer response, e.g. "Lex is a jerk", it doesn't spot it at all.
it seems to be the .contains that isn't working. I have seen people in the forums with arrays etc, but none of the tutorials or teachers notes or even the notes in the extra credit section mention that Arrays should be brought into it (are people just going a little further than they need to, or is Arrays the actual solution?).
Thanks in advance, I've literally been trying to figure this out for 2 hours now :(
import java.io.Console;
public class TreeStory {
public static void main(String[] args) {
Console console = System.console();
String ageAsString = console.readLine("How old are you?\n");
int age = Integer.parseInt(ageAsString);;
if (age < 13) {
//insert exit code
console.printf("Sorry, you must be at least 13 to use this program.\n");
System.exit(0);
}
//Time to make changes here. This is where I incorporate the "contains" method. Or attempt to.
String name = console.readLine("Enter a name: ");
String adjective = console.readLine("Enter an adjective: ");
String noun;
boolean isInvalidWord;
String areBadWords = "dork jerk nerd geek numpty";
do {
noun = console.readLine("Enter a noun: ");
isInvalidWord = (areBadWords.contains(noun.toLowerCase()));
if (isInvalidWord) {
console.printf("That language is not allowed. Try again. \n\n");
}
} while(isInvalidWord);
//This is the end of my changes
String adverb = console.readLine("Enter an adverb: ");
String verb = console.readLine("Enter a verb ending with -ing: ");
console.printf("Your TreeStory: \n---------------\n");
console.printf("%s is a %s %s. They are always %s %s.", name, adjective, noun, adverb, verb);
}
}
2 Answers
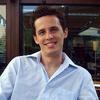
Pedro Cabral
33,586 PointsIt doesn't work because the string "dork jerk nerd geek numpty" doesn't contain "Lex is a jerk". You need a loop, for every String in the answer, if the bad words contain that String then you can flag it:
for (String word : noun.split(" ")) { // rest of code }
or you could also not allow the noun to be more than one word, by extracting the first String with: readLine().split(" ")[0]
I think both approaches would work, and probably the last one would make more sense. Since the var is called noun, why would you allow a sentence in the first place?

Ken Alger
Treehouse TeacherOlexa;
The method we are using in this task is the String.contains method. As Pedro mentions, this method will return true if and only if this string contains the specified sequence of char values.
Post back with further questions.
Ken
Lex Dunko
Data Analysis Techdegree Student 4,449 PointsLex Dunko
Data Analysis Techdegree Student 4,449 PointsThanks for your help Pedro. My understanding of contain was if the noun contained any of my words in String areBadWords, it would flag.
My preference would be to use your first example (no offence to your second one, I just think the first one is where my curiosity lies). Where about would I place this in my code? Sorry if I'm asking to be spoon fed here, still getting to grips with it - total newbie.
This is what I tried, but didn't work (albeit no errors):