Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial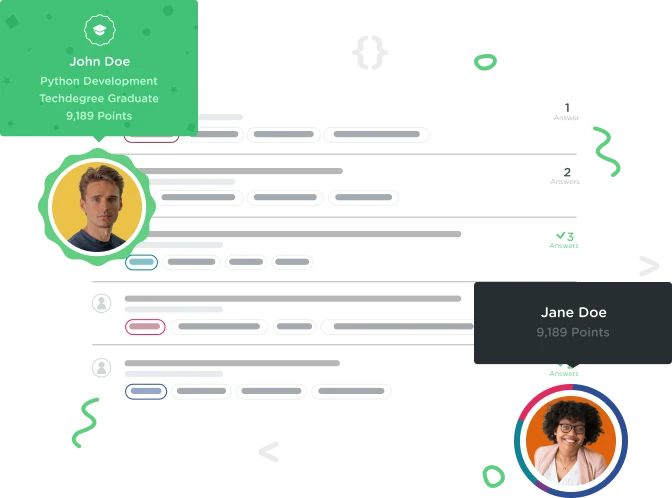

Alexander Bellantine
Courses Plus Student 545 PointsTreeStory Expansion problem
Dear TReeHouse community,
After we finished the java basics track, we left a prototype of a project for the TreeStory program. I've been growing the project and adding different classes to the project to handle different problems like censoring and administrative access. However, in creating the "censor module", I only have two more errors to fix. but no matter how hard I try or research, I can't get the (reached end of file while parsing ' } ' ) on line 56 or the (reached end of file while parsing) on 57. Thank you very much! Here's the code:
public class CensorModule {
// holds word used String word; // represents good word String goodWord; // represents bad word String badWord; // placeholders until project finished String name; String admin;
// define what is a good word and a bad word
public String censorDatabase() {
if (word.contains("fuck") ||
word.contains("Fuck") ||
word.contains("Shit") ||
word.contains("shit") ||
word.contains("Pussy") ||
word.contains("pussy") ||
word.contains("Dick") ||
word.contains("dick") ||
word.contains("Bitch") ||
word.contains("bitch") ||
word.contains("Cunt") ||
word.contains("cunt")){
word = badWord;
}
else {
word = goodWord;
}
}
// loop that restricts bad words and allows good words, unless user is an admin in which all words are allowed public String censorModule() { boolean badWordDetected; do { if (word == goodWord) { return word; badWordDetected = false; } if (word == badWord) { System.out.printf("That's not nice! Try again "); badWordDetected = true; } if (word == badWord && name == admin){ return word; badWordDetected = false; } } while (badWordDetected = true); return } }
1 Answer
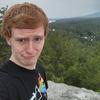
Brendon Butler
4,254 Pointspublic class CensorModule {
String word; // word variable was never assigned
String goodWord;
String badWord;
String name;
String admin;
// censoredDatabase is never explicitly used
public String censorDatabase() {
if (word.contains("...") || word.contains("...") || word.contains("...") || word.contains("...")) { // this is a poorly optimized way to process your strings
word = badWord; // this does nothing as the badWord variable was never assigned.
} else {
word = goodWord; // this does nothing as the goodWord variable was never assigned.
}
}
public String censorModule() {
boolean badWordDetected; // this should be false by default
do {
if (word == goodWord) {
return word;
badWordDetected = false; // this line will never be processed since you have a return statement above it. You should have this line before your return line
}
if (word == badWord) {
System.out.printf("That's not nice! Try again ");
badWordDetected = true;
}
if (word == badWord && name == admin) { // you should have this if block before your second if block, since if an admin says a bad word, it will print "That's not nice! Try again ". Then, it will still accept the user's input.
return word;
badWordDetected = false; // this line will never be processed since you have a return statement above it. You
should have this line before your return line
}
} while (badWordDetected = true);
return // you're not returning anything and you
}
}
I would love to show you how to fix what you have, but it's not worth it. So here's a better way of doing what you want to do:
public class CensorModule {
private String[] badWords = {"poop", "fart", "idiot"}; // put your profanity here in lowercase, in quotes, divided by commas
public static boolean containsBadWord(String input) {
for (String word : badWords)
if (input.toLowerCase().contains(word)
return true;
return false;
}
}
Then you can use this in another class by doing this:
boolean profanity = false;
String input;
do {
System.out.println("Write a sentence: ");
input = console.readLine();
if (CensorModule.containsBadWord(input)) {
profanity = true;
System.out.printf("That's not nice! Try again.%n%n%n");
}
} while (profanity);
// if there aren't any bad words used, you can then use the input string here after the loop.
This isn't a perfect system, there are ways to get around the checker. But this is a good start here. If you have any questions. Please ask.
Alexander Bellantine
Courses Plus Student 545 PointsAlexander Bellantine
Courses Plus Student 545 PointsThank you so much! It's awesome to know there's a community of programmers willing to help with stuff like this