Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial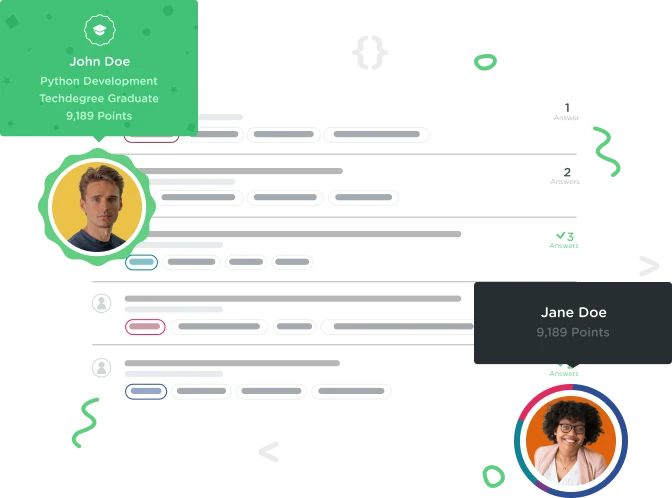

Josh Keenan
20,315 Points[SOLVED]Access private variables in a subclass
EDIT: Realised I was using the wrong name, I was using the parameter name and not the variable where I was storing stuff, but this has shown me that rubber ducks are the best idea ever, talking to myself and anyone who reads this helped, so thanks for reading <3
Apologies as that may not be the right phrasing but I'm brand new to java, which is making this debugging all the harder.
I am trying to calculate the interest for an imaginary savings account but I am unable to access bal in the SavingsAccount, can someone help me out and explain what's wrong? Many thanks <3
FYI there are different methods I was trying to use to get this to work, which I have left in this snippet, so I am aware they are there and not right or the same, I am a novice Java programmer trying different things I can think of to get this to work!
public class BankAccount {
private int balance;
private int accountNumber;
private String holder;
// Constructor
public BankAccount(int id, String name, int bal)
{
accountNumber = id;
holder = name;
balance = bal;
}
public String toString()
{
return String.format("Account number: %d\nAccount holder: %s\n" +
"Account balance: %d\n", accountNumber, holder,
balance);
}
// method to deposit into account, checks amount is valid returns bool
public void deposit(int amount)
{
try
{
balance += amount;
System.out.format("Deposited £%d\n", amount);
}
catch (ArithmeticException e)
{
System.out.println("Invalid amount\n");
}
}
// method to withdraw from account
public void withdraw(int amount)
{
try
{
balance -= amount;
System.out.format("Withdrawn £%d\n", amount);
}
catch (ArithmeticException e)
{
System.out.println("Insufficient Funds!\n");
}
}
// getter to return id
public int getId()
{
System.out.println("Account number: ");
return accountNumber;
}
// getter to return account holder name
public String getName()
{
System.out.println("Account holder: ");
return holder;
}
public int getBalance()
{
System.out.println("Account balance: £");
return balance;
}
// public static void main(String[] args)
// {
//
// BankAccount account = new BankAccount(233, "John", 55);
// account.deposit(500);
// account.withdraw(50);
//
// System.out.println(account.getId() + "\n");
// System.out.println(account.getBalance() + "\n");
// }
} // The end.
import java.lang.Math;
public class SavingsAccount extends BankAccount {
private int rate;
private int storageVar;
// Constructor
public SavingsAccount(int id, String name, int bal, int val)
{
super(id, name, bal);
rate = val;
}
public int getRate()
{
return rate;
}
public int setRate(int newRate)
{
rate = newRate;
return rate;
}
public int rateCalculator()
{
storageVar = bal * (1 + (rate / 100));
System.out.format("Balance in 1 year: £%d\n", storageVar);
storageVar = this.bal * Math.pow((1 + (rate / 100)), 5);
System.out.format("Balance in 5 years: £%d\n", storageVar);
storageVar = this.bal * Math.pow((1 + (rate / 100)), 10);
System.out.format("Balance in 10 years: £%d\n", storageVar);
}
}
1 Answer

Josh Keenan
20,315 PointsError being thrown:
SavingsAccount.java:31: error: cannot find symbol
storageVar = bal * (1 + (rate / 100));
^
symbol: variable bal
location: class SavingsAccount
SavingsAccount.java:33: error: cannot find symbol
storageVar = bal * Math.pow((1 + (rate / 100)), 5);
^
symbol: variable bal
location: class SavingsAccount
SavingsAccount.java:35: error: cannot find symbol
storageVar = this.bal * Math.pow((1 + (rate / 100)), 10);
^
symbol: variable bal
Josh Keenan
20,315 PointsJosh Keenan
20,315 Pointsapologies some formatting has broken on transfer from IDE to treehouse! Will try to fix it