Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial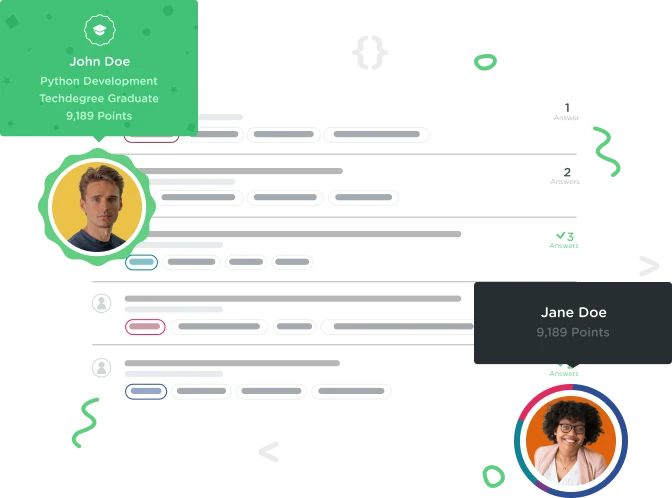

Jean Malan
10,781 PointsRuby problem not compiling properly!
Hey guys!
Im suppose to run this code where the user gets a prompt to input the rover position (which will be 0,0) and then a set of instructions to manoeuvre the rover which will be: up 5, down 3, left 3, right 2, up 2, left 1. This is then stored in an array like:
[“UP 5”, “DOWN 3”, “LEFT 3”, “RIGHT 2”, “UP 2”, “LEFT 1”]
However the output I receive is x = 0, y =1 but it should be x = 0, y = 4. I have no idea where the equation is going wrong?! Any help please :)
class MarsRover
attr_accessor :position,:behaviour,:x,:y
end
# input x & y positions
def rover_position
@position = {}
puts "\nPlease input the first rover's X position: "
@position["x"] = gets.chomp.to_i
puts "\nPlease input the first rover's Y position: "
@position["y"] = gets.chomp.to_i
end
def rover_behaviour
puts " \n Please input the behaviour of the first rover (eg: Down 1, Up 2, Left 1, Right 3): "
input = gets.chomp.upcase
@behaviour = input.split(', ')
@behaviour.each do |behavior|
case behavior
when 'DOWN 1', 'DOWN 2', 'DOWN 3', 'DOWN 4', 'DOWN 5', 'DOWN 6', 'DOWN 7',
'DOWN 8', 'DOWN 9', 'DOWN 10'
move_down
when 'UP 1', 'UP 2', 'UP 3', 'UP 4', 'UP 5', 'UP 6', 'UP 7', 'UP 8',
'UP 9', 'UP 10'
move_up
when 'LEFT 1', 'LEFT 2', 'LEFT 3', 'LEFT 4', 'LEFT 5', 'LEFT 6', 'LEFT 7',
'LEFT 8', 'LEFT 9', 'LEFT 10'
move_left
when 'RIGHT 1', 'RIGHT 2', 'RIGHT 3', 'RIGHT 4', 'RIGHT 5', 'RIGHT 6',
'RIGHT 7', 'RIGHT 8', 'RIGHT 9', 'RIGHT 10'
move_right
end
end
end
# move rover left as per behaviour input
def move_down
case
when
@behaviour.include?('DOWN 1')
@position["y"] -= 1
when
@behaviour.include?('DOWN 2')
@position["y"] -= 2
when
@behaviour.include?('DOWN 3')
@position["y"] -= 3
when
@behaviour.include?('DOWN 4')
@position["y"] -= 4
when
@behaviour.include?('DOWN 5')
@position["y"] -= 5
when
@behaviour.include?('DOWN 6')
@position["y"] -= 6
when
@behaviour.include?('DOWN 7')
@position["y"] -= 7
when
@behaviour.include?('DOWN 8')
@position["y"] -= 8
when
@behaviour.include?('DOWN 9')
@position["y"] -= 9
when
@behaviour.include?('DOWN 10')
@position["y"] -= 10
end
end
def move_up
case
when
@behaviour.include?('UP 1')
@position["y"] += 1
when
@behaviour.include?('UP 2')
@position["y"] += 2
when
@behaviour.include?('UP 3')
@position["y"] += 3
when
@behaviour.include?('UP 4')
@position["y"] += 4
when
@behaviour.include?('UP 5')
@position["y"] += 5
when
@behaviour.include?('UP 6')
@position["y"] += 6
when
@behaviour.include?('UP 7')
@position["y"] += 7
when
@behaviour.include?('UP 8')
@position["y"] += 8
when
@behaviour.include?('UP 9')
@position["y"] += 9
when
@behaviour.include?('UP 10')
@position["y"] += 10
end
end
def move_right
case when
@behaviour.include?('RIGHT 1')
@position["x"] += 1
when
@behaviour.include?('RIGHT 2')
@position["x"] += 2
when
@behaviour.include?('RIGHT 3')
@position["x"] += 3
when
@behaviour.include?('RIGHT 4')
@position["x"] += 4
when
@behaviour.include?('RIGHT 5')
@position["x"] += 5
when
@behaviour.include?('RIGHT 6')
@position["x"] += 6
when
@behaviour.include?('RIGHT 7')
@position["x"] += 7
when
@behaviour.include?('RIGHT 8')
@position["x"] += 8
when
@behaviour.include?('RIGHT 9')
@position["x"] += 9
when
@behaviour.include?('RIGHT 10')
@position["x"] += 10
end
end
def move_left
case when
@behaviour.include?('LEFT 1')
@position["x"] -= 1
when
@behaviour.include?('LEFT 2')
@position["x"] -= 2
when
@behaviour.include?('LEFT 3')
@position["x"] -= 3
when
@behaviour.include?('LEFT 4')
@position["x"] -= 4
when
@behaviour.include?('LEFT 5')
@position["x"] -= 5
when
@behaviour.include?('LEFT 6')
@position["x"] -= 6
when
@behaviour.include?('LEFT 7')
@position["x"] -= 7
when
@behaviour.include?('LEFT 8')
@position["x"] -= 8
when
@behaviour.include?('LEFT 9')
@position["x"] -= 9
when
@behaviour.include?('LEFT 10')
@position["x"] -= 10
end
end
def final_position()
seporator2 = "\n"
seporator = "-"
seporator2 = "\n"
puts seporator * 80
puts seporator2
puts "\n The position of the first rover is #{@position} \n"
puts seporator * 80
puts seporator2
end
print rover_position
print rover_behaviour
print final_position
run_rover1 = MarsRover.new
1 Answer

Jack Foo
2,728 PointsHi, would like to answer your question.
From the method rover_behavior you have an iteration of @behavior.each do |behavior| block, and inside the block you are calling the method move_up/down/left/right depending on the variable |behavior| which is the element inside the @behavior array, but when you call the method move_up/down/left/right, you are calling @behavior.include? which is checking for the whole array (not the element).
So at rover_behavior you are checking for @behavior array element; and at move_up/down/left/right you are checking for @behavior the whole array.
Therefore even though in the method_behavior @behavior.each do block you are iterating the variable |behavior| "left 3", but when calling the method move_left it will still run @position['x'] -= 1 because it will check if @behavior includes "left 1" first, so ignoring the "left 3".
Use this instead:
def rover_behaviour
puts " \nPlease input the behaviour of the first rover (eg: Down 1, Up 2, Left 1, Right 3): "
input = gets.chomp.upcase
@behaviour = input.split(', ')
@behaviour.each do |behavior|
direction, movement = behavior.split(" ")
movement = movement.to_i
case direction
when 'DOWN'
@position["y"] -= movement
when 'UP'
@position["y"] += movement
when 'LEFT'
@position["x"] -= movement
when 'RIGHT'
@position["x"] += movement
end
end
end
By the way you are not using the class and defining class methods & instance variables correctly, I suggest you can revisit the course and learn more about how to properly define class and use it.
Hope you find the above useful.