Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial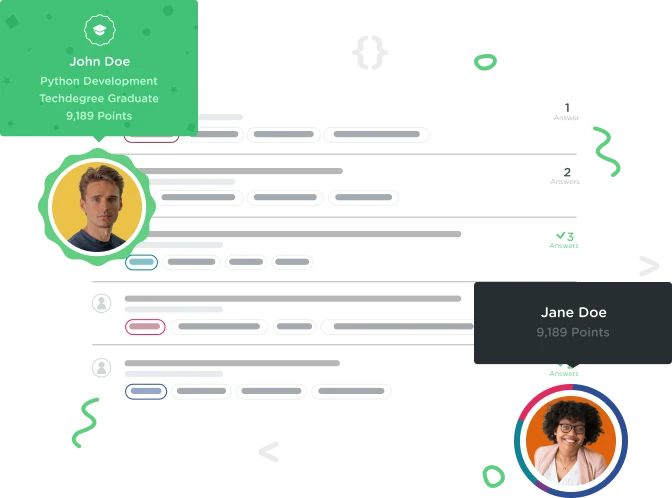

senenge iyortyom
30 Pointsprocessing
.Q1: A Stationary VehicleYou should be able to do this question after Week 2 in the course.Write a non-active Processing program (containing no setup() ordraw() functions) which will draw some sort of vehicle in the canvas. Itcan be anything you like: a car, a boat, a plane, a skateboard, a pogostick, or anything else that can move something from one place toanother. Be creative. The rules are:●It must be a real-world vehicle. No Starships or Tardises, please.●It must contain from 6 to 16 shapes (lines, ellipses, rectangles, orother shapes). The sample ship shown here uses 9 shapes (2 rectangles, 3 ellipses, 2triangles, and 2 lines).●It must contain at least one line, at least one ellipse or circle, and at least one square,rectangle, triangle, or quadrilateral (quad).●It should contain several different colours.●It can be a very abstract vehicle, like the one shown. You don’t have to be a great artist.●Don’t make it too complex. Stick to the limit of 16 shapes. That will make the remainingquestions easier.●You must use a 500 x 500 canvas for this question.●The vehicle must fill the canvas, either horizontally (as the ship does), or vertically.●It should be roughly centred in the window.In Questions 2 and 3, your vehicle will grow, shrink, and move. As soon as any program is“finished”, you usually have to start making changes to it, and that will happen with thisprogram, too. To make that easier to do, you must do the following things:●All X coordinates, Y coordinates, heights, widths, and colours, must be defined byconstants. You will probably need at least 10, and perhaps as many as 30 if you choose todo a very complex vehicle.1 ASSIGNMENT 1DEPARTMENT AND COURSE NUMBER: COMP 1010●The X and Y coordinates should be specified relative to the centre of the window. Thiswill make the following questions much easier. Heights and widths will be in pixels. Forexample, these 2 constants were used to define parts of the example ship. The Ycoordinate -50 means the top of the ship is 50 pixels above the centre of the canvas.final int DECK_HEIGHT_Y=-50; //Y coordinate of the top of the shipfinal int BODY_WIDTH=300; //total width of rectangular body section●All of your drawing commands should use your constants, the built-in height andwidth variables, and perhaps simple numbers like 1 or 2. No other numbers. Forexample, the rectangle that forms the main body of the ship was drawn with the statementbelow.rect(width/2-BODY_WIDTH/2, height/2+DECK_HEIGHT_Y, BODY_WIDTH, BODY_HEIGHT);●If some calculation is needed many times in many statements, do it only once and storethe result in a variable. Then use that variable many times. For example, a better versionof the above statement would be the one below, where centreX and shipTop werepre-calculated, since those values were needed many times to draw the entire ship. (Hint:It would be a really good idea to pre-calculate the coordinates of the centre of thecanvas.)rect(centreX-BODY_WIDTH/2,shipTop,BODY_WIDTH,BODY_HEIGHT);
Q2: A Moving VehicleThis question requires material from Week 3.Convert your program from Question 1 into an ActiveProcessing program, with the mouse controlling both the sizeand position of the vehicle.Make the following changes and additions to your Question 1program.1.Save a copy of your original Question 1 program. Youmust hand in the original static version, as well as thismodified active version. Rename this one so that it endswith “A1Q2”.2.Add three variables to your program, which control the vehicle’s size and position. Thereshould be an X coordinate, a Y coordinate, and a size value. The X and Y coordinatesshould specify the position of the approximate centre of the vehicle (the same spot thatused to be at the centre of the canvas, but could now be anywhere). The size variableshould give the total width or height of the vehicle (which used to be exactly 500 but nowcould be any size).3.Create the usual setup()and draw() functions. Create a drawVehicle() function (orcall it drawShip() or drawSkateboard(), or whatever name is appropriate for you).Create a moveVehicle() function (or use a similar name).4.Move all of your existing code from Question 1 into the appropriate place in these newfunctions. All of the code that draws your vehicle should go into the drawVehicle()2 ASSIGNMENT 1DEPARTMENT AND COURSE NUMBER: COMP 1010function, not into the draw() function directly. The functions should call each other asneeded.5.The moveVehicle() function should use the mouse position to set the X, Y, and sizevalues for your vehicle (the ones described in point 2). The X and Y values shouldexactly match the mouse position. The size value should match the mouseY value, so thatyour vehicle will be drawn full-size at the bottom of the canvas, and have size 0 at the topof the canvas (and therefore disappear entirely). This will be a very short function.6.Now you need to change all of the commands that draw your vehicle, so that they takeinto account its position and size. If you took the hint in Question 1, controlling theposition should be very easy. To control the size, you will have to “scale” almost all ofthe constants from Question 1, using the vehicle’s current size, and its original size (500).For example, BODY_HEIGHT used to control the height of the example ship’s body. Nowit must be BODY_HEIGHT*shipSize/ORIGINAL_SIZE. (You were planning to use aname, and not just the number 500. Right?)7.When using int values, be careful. BODY_HEIGHT*shipSize/ORIGINAL_SIZE worksbut shipSize/ORIGINAL_SIZE*BODY_HEIGHT will usually just give you 0. Alwaysdo the multiplication first. And don’t try to store shipSize/ORIGINAL_SIZE in an intvariable, as nice as that would be. You’ll just get 0.8.Now change the size of the canvas to 1000 by 1000 pixels, or to any other size. If you’vewritten your program well, everything should still work, with no modification. Did it?
Q3: A Self-propelled VehicleConvert your program from Question 2 so that your vehicle will move across the canvas, andchange size, all by itself. Here’s how it should work: When you click the mouse in the canvas,your vehicle should slowly and smoothly move to that position (or close to it).Make the following changes and additions to your Question 2 program.1.Save a copy of your original Question 2 program. You must hand in that version, as wellas this new version. Rename this one so that it ends with “A1Q3”.2.Add a mouseClicked() function (void mouseClicked()). This is a specialfunction, like setup() and draw(), which is automatically called every time there is amouse click in the canvas. The mouseX and mouseY variables give the location of theclick. The location of the last mouse click should be stored in appropriately-named statevariables.3.Change your moveVehicle() function (or whatever you have chosen to name it) so thatyour vehicle will now move slowly and steadily toward the location of the most recentmouse click. Use a constant to control your vehicle’s speed. In each frame, it shouldmove 1/Nth of the way from its current position to the location of the mouse click. (Butdon’t call the constant N – use a better name.) Note: When the vehicle gets close to thedesired location, it will reach a point where its position will change by less than 1 pixelper frame. Since you are using int variables, you will get a change of 0 pixels per frame.
Q4:..Add some sort of animation to your vehicle. One or more of the shapes that make up thevehicle should change in some way, in a repeating pattern. Some part of your vehiclecould move up and down, or rotate, or change in size, or whatever you like. It should be arepeating animation that can run indefinitely. It can be something realistic, or a“cartoonish” animation that is unusual.3.Add appropriate constants and state variables to your program to control the animation.4.Add an animateVehicle() function, or a function with a similar name, to control theanimation.5.Change the drawVehicle() function, or whatever you have named it, so that itperforms the drawing in this new way. (There should be no drawing commands.
2 Answers
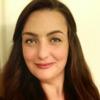
Jennifer Nordell
Treehouse TeacherHi there! The Community is a great place to get help even on topics not specifically regarding Treehouse material. However, in order to get an answer, it is generally advised to first post a question. Your "question" actually contains no actual question but rather a list of specifications for a project that does not seem to exist inside the Treehouse curriculum.
I would suggest that if you sincerely want help with this, that you first post a link to a repository where people who are familiar with this type of project can review your code and potentially help you debug it. Short of doing this we really have no way to help you, nor any desire to do your project for you. Ultimately, you are responsible for the outcome of your project.
Good luck!
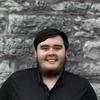
Michael Hulet
47,913 PointsTo add onto this, "Please do my homework for me, here are the specifications" isn't a question appropriate for this forum. You'll need to narrow it down to a single, specific question about what you're specifically having trouble with

senenge iyortyom
30 Pointsthats the actual question
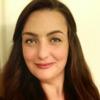
Jennifer Nordell
Treehouse TeacherThat's an assignment and a list of specifications. It is not a question
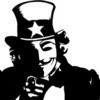
behar
10,799 PointsIt's a great question! The sun is shining?
behar
10,799 Pointsbehar
10,799 PointsWay too lazy to read thru all of this, do you have an actual question?