Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial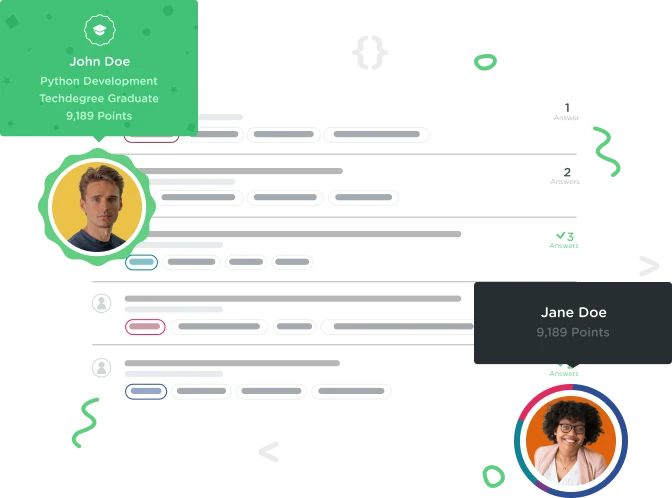
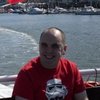
Sean Flanagan
33,236 PointsProblems with KaraokeMachine
Hi. My KaraokeMachine has generated a ton of errors. Here it is:
package com.teamtreehouse;
import com.teamtreehouse.model.Song;
import com.teamtreehouse.model.SongBook;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.HashMap;
import java.util.Map;
public class KaraokeMachine {
private SongBook mSongBook;
private BufferedReader mReader;
private Map<String, String> mMenu;
public KaraokeMachine(SongBook songBook) {
mSongBook = songBook;
mReader = new BufferedReader(new InputStreamReader(System.in));
mMenu = new HashMap<String, String>();
mMenu.put("add", "Add a new song to the song book");
mMenu.put("quit", "Give up. Exit the program");
}
private String promptAction() throws IOException {
System.out.printf("There are %d songs available. Your options are: %n",
mSongBook.getSongCount());
for (Map.Entry<String, String> option : mMenu.entrySet()) {
System.out.printf("%s - %s %n",
option.getKey(),
option.getValue());
}
System.out.print("What do you want to do: ");
String choice = mReader.readLine();
return choice.trim().toLowerCase();
}
public void run() {
String choice="";
do {
try {
choice = promptAction();
switch(choice) {
case "add":
Song song = promptNewSong();
mSongBook.addSong(song);
System.out.printf("%s added! %n%n", song);
break;
case "quit":
System.out.println("Thanks for playing!");
break;
default:
System.out.printf("Unknown choice: '%s'. Try again. %n%n%n",
choice);
}
} catch(IOException ioe) {
System.out.println("Problem with input");
ioe.printStackTrace();
}
} while (!choice.equals("quit"));
}
private Song promptNewSong() throws IOException {
System.out.print("Enter the artist's name: ");
String.artist = mReader.readLine();
System.out.print("Enter the title: ");
String.title = mReader.readLine();
System.out.print("Enter the video URL: ");
String.videoUrl = mReader.readLine();
return new Song(artist, title, videoUrl);
}
}
Next, I'll list the errors:
./com/teamtreehouse/KaraokeMachine.java:61: error: while expected
}
^
./com/teamtreehouse/KaraokeMachine.java:63: error: ')' expected
private Song promptNewSong() {
^
./com/teamtreehouse/KaraokeMachine.java:63: error: -> expected
private Song promptNewSong() {
^
./com/teamtreehouse/KaraokeMachine.java:63: error: not a statement
private Song promptNewSong() {
^
./com/teamtreehouse/KaraokeMachine.java:65: error: cannot find symbol
String.artist = mReader.readLine();
^
./com/teamtreehouse/KaraokeMachine.java:65: error: cannot find symbol
String.artist = mReader.readLine();
^
symbol: variable artist
location: class String
./com/teamtreehouse/KaraokeMachine.java:67: error: cannot find symbol
String.title = mReader.readLine();
^
symbol: variable title
location: class String
./com/teamtreehouse/KaraokeMachine.java:69: error: cannot find symbol
String.videoUrl = mReader.readLine();
^
symbol: variable videoUrl
location: class String
./com/teamtreehouse/KaraokeMachine.java:70: error: cannot find symbol
return new Song(artist, title, videoUrl);
treehouse:~/workspace$
I'd be grateful for any help. :-)
3 Answers

Craig Dennis
Treehouse TeacherLooks like promptNewSong
is outside of your class. Correct the try
to have the catch
right after it. You are missing a closing paren.
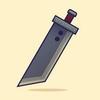
Allan Clark
10,810 Points } catch(IOException ioe) {
System.out.println("Problem with input");
ioe.printStackTrace();
} // <-------- this one
} while (!choice.equals("quit"));
}
It looks like you have too many '}' try deleting the one I marked and running it again. The hint from the error list "error: while expected }" is telling you that the do-while loop was closed but it didn't find the while part.
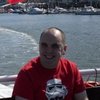
Sean Flanagan
33,236 PointsHi Allan. Thanks for your reply.
I deleted said } but the program still won't run.
Here's what I have now:
package com.teamtreehouse;
import com.teamtreehouse.model.Song;
import com.teamtreehouse.model.SongBook;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.HashMap;
import java.util.Map;
public class KaraokeMachine {
private SongBook mSongBook;
private BufferedReader mReader;
private Map<String, String> mMenu;
public KaraokeMachine(SongBook songBook) {
mSongBook = songBook;
mReader = new BufferedReader(new InputStreamReader(System.in));
mMenu = new HashMap<String, String>();
mMenu.put("add", "Add a new song to the song book");
mMenu.put("quit", "Give up. Exit the program");
}
private String promptAction() throws IOException {
System.out.printf("There are %d songs available. Your options are: %n",
mSongBook.getSongCount());
for (Map.Entry<String, String> option : mMenu.entrySet()) {
System.out.printf("%s - %s %n",
option.getKey(),
option.getValue());
}
System.out.print("What do you want to do: ");
String choice = mReader.readLine();
return choice.trim().toLowerCase();
}
public void run() {
String choice="";
do {
try {
choice = promptAction();
switch(choice) {
case "add":
Song song = promptNewSong();
mSongBook.addSong(song);
System.out.printf("%s added! %n%n", song);
break;
case "quit":
System.out.println("Thanks for playing!");
break;
default:
System.out.printf("Unknown choice: '%s'. Try again. %n%n%n",
choice);
}
} catch(IOException ioe) {
System.out.println("Problem with input");
ioe.printStackTrace();
} while (!choice.equals("quit"));
}
private Song promptNewSong() throws IOException {
System.out.print("Enter the artist's name: ");
String.artist = mReader.readLine();
System.out.print("Enter the title: ");
String.title = mReader.readLine();
System.out.print("Enter the video URL: ");
String.videoUrl = mReader.readLine();
return new Song(artist, title, videoUrl);
}
}
And the errors:
./com/teamtreehouse/KaraokeMachine.java:72: error: reached end of file while parsing
}
^
./com/teamtreehouse/KaraokeMachine.java:45: error: cannot find symbol
Song song = promptNewSong();
^
symbol: method promptNewSong()
location: class KaraokeMachine
./com/teamtreehouse/KaraokeMachine.java:63: error: cannot find symbol
private Song promptNewSong() throws IOException {
^
symbol: variable Song
location: class KaraokeMachine
./com/teamtreehouse/KaraokeMachine.java:63: error: illegal start of type
private Song promptNewSong() throws IOException {
^
./com/teamtreehouse/KaraokeMachine.java:63: error: lambda expression not expected here
private Song promptNewSong() throws IOException {
^
./com/teamtreehouse/KaraokeMachine.java:65: error: cannot find symbol
String.artist = mReader.readLine();
^
symbol: variable artist
location: class String
./com/teamtreehouse/KaraokeMachine.java:67: error: cannot find symbol
String.title = mReader.readLine();
^
symbol: variable title
location: class String
./com/teamtreehouse/KaraokeMachine.java:69: error: cannot find symbol
String.videoUrl = mReader.readLine();
^
symbol: variable videoUrl
location: class String
I'm not sure what else to do. Thanks for your help though. :-)
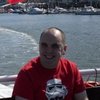
Sean Flanagan
33,236 PointsHere's my KaraokeMachine as it presently stands:
package com.teamtreehouse;
import com.teamtreehouse.model.Song;
import com.teamtreehouse.model.SongBook;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.HashMap;
import java.util.Map;
public class KaraokeMachine {
private SongBook mSongBook;
private BufferedReader mReader;
private Map<String, String> mMenu;
public KaraokeMachine(SongBook songBook) {
mSongBook = songBook;
mReader = new BufferedReader(new InputStreamReader(System.in));
mMenu = new HashMap<String, String>();
mMenu.put("add", "Add a new song to the song book");
mMenu.put("quit", "Give up. Exit the program");
}
private String promptAction() throws IOException {
System.out.printf("There are %d songs available. Your options are: %n",
mSongBook.getSongCount());
for (Map.Entry<String, String> option : mMenu.entrySet()) {
System.out.printf("%s - %s %n",
option.getKey(),
option.getValue());
}
System.out.print("What do you want to do: ");
String choice = mReader.readLine();
return choice.trim().toLowerCase();
}
public void run() {
String choice="";
do {
try {
choice = promptAction();
switch(choice) {
case "add":
Song song = promptNewSong();
mSongBook.addSong(song);
System.out.printf("%s added! %n%n", song);
break;
case "quit":
System.out.println("Thanks for playing!");
break;
default:
System.out.printf("Unknown choice: '%s'. Try again. %n%n%n",
choice);
}
} catch(IOException ioe) {
System.out.println("Problem with input");
ioe.printStackTrace();
}
} while (!choice.equals("quit"));
}
private Song promptNewSong() throws IOException {
System.out.print("Enter the artist's name: ");
String.artist = mReader.readLine();
System.out.print("Enter the title: ");
String.title = mReader.readLine();
System.out.print("Enter the video URL: ");
String.videoUrl = mReader.readLine();
return new Song(artist, title, videoUrl);
}
}
And the errors:
^
symbol: variable artist
location: class String
./com/teamtreehouse/KaraokeMachine.java:67: error: cannot find symbol
String.title = mReader.readLine();
^
symbol: variable title
location: class String
./com/teamtreehouse/KaraokeMachine.java:69: error: cannot find symbol
String.videoUrl = mReader.readLine();
^
symbol: variable videoUrl
location: class String
./com/teamtreehouse/KaraokeMachine.java:70: error: cannot find symbol
return new Song(artist, title, videoUrl);
^
symbol: variable artist
location: class KaraokeMachine
./com/teamtreehouse/KaraokeMachine.java:70: error: cannot find symbol
return new Song(artist, title, videoUrl);
^
symbol: variable title
location: class KaraokeMachine
./com/teamtreehouse/KaraokeMachine.java:70: error: cannot find symbol
return new Song(artist, title, videoUrl);
^
symbol: variable videoUrl
location: class KaraokeMachine
6 errors

Craig Dennis
Treehouse TeacherThere seem to be dots between your type declaration and variable name. The String.title
error, that's not what you meant is it?
Sean Flanagan
33,236 PointsSean Flanagan
33,236 PointsHi Craig. Thanks for replying. I've added the closing parenthesis. But I'm confused about the rest. When you say I should correct the try to have the catch right after it, do you mean I should type:
It's just that I'm still getting errors.
Sean Flanagan
33,236 PointsSean Flanagan
33,236 PointsHi Craig. Just to let you know I've fixed the errors. Near the end of my KaraokeMachine class were 3 errors, each involving a dot that shouldn't have been there, between String and artist, between String and title, and between String and videoUrl. I've deleted all the dots and my program is now running. Thank to you and Allan for your help. :-)