Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial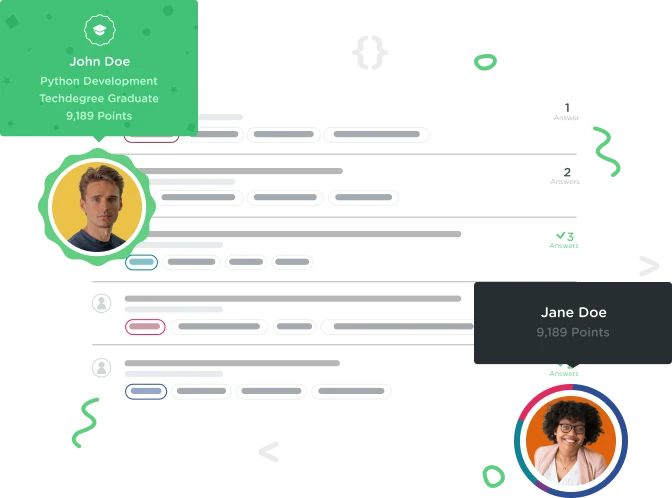
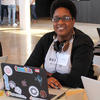
Rachél Bazelais
10,583 PointsMystery Uncaught TypeError: Cannot read property 'children' of null
I have no idea what's wrong. Can someone help me understand this error and why i'm getting it?
Here's my code:
//Problem: User interaction doesn't provide desired results.
//Solution: Add interactivity so the user can manage daily tasks.
var taskInput = document.getElementById("new-tasks"); //new-tasks
var addButton = document.getElementsByTagName("button")[0]; //first button
var incompleteTasksHolder = document.getElementById("incompleted-tasks"); //incompleted-tasks
var completedTasksHolder = document.getElementById("completed-tasks"); //completed-tasks
//add a new task
var addTask = function(){
console.log("Add Task...");
//when a button is pressed create a new task
//create a new list item with the text from #new-task:
//create input with a checkbox
//input (checkbox)
//label
//input (text)
//button.edit
//button.delete
//Each element has to be modified and appended
}
//edit an existing task
var editTask = function(){
console.log("Edit Task...");
//if the class of the parent is in .editMode
//switch from .editMode
//the label text becomes input's the value
//else
//switch to .editMode
//the label value becomes label's the text
//toggle .editMode on the parent
}
//delete an existing task
var deleteTask = function() {
console.log("Delete Task...");
//when the delete button is pressed
//remove the parent (li) list item from the (ul)unordered list
}
//mark a task as complete
var taskCompleted = function(){
console.log("Task Complete ...");
//when checkbox is checked
//append the task list item to the #completed-tasks
}
//mark a task as incomplete
var taskIncomplete = function(){
console.log("Task Incomplete...");
//when checkbox is unchecked
//append the task list item to the #incompleted-tasks
}
var bindTaskEvents = function(taskListItem, checkboxEventHandler){
console.log("Bind list item events");
//select taskListItem's children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind editTask to edit button
editButton.onclick = editTask;
//bind deleteTask to the delete button
deleteButton.onclick = deleteTask;
//bind checkboxEventHandler to checkbox
checkBox.onchange = checkBoxEventHandler;
}
//set the click handler to the add task function
//call the function when the user interacts with the button
addButton.onlick = addTask;
//cycle over incompleteTaskHolder ul list item
for (var i = 0; i < incompleteTasksHolder.children.length; i++){
//bind events to list item's children (taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted);
}
//cycle over completeTaskHolder ul list item
for (var i = 0; i < CompletedTasksHolder.children.length; i++){
//bind events to list item's children (taskIncomplete);
bindTaskEvents(CompletedTasksHolder.children[i], taskIncomplete);
}
1 Answer
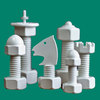
Steven Parker
241,958 Points
You have a capitalization mismatch.
On the last few lines, you reference "CompletedTasksHolder" twice, but your variable name established at the top is "completedTasksHolder" (lower case "c").
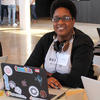
Rachél Bazelais
10,583 PointsThanks for reponding! I fixed the variable name but i'm still getting the same error. Specifically on line 79 but there's no syntax error
Snippet: Line 79: for (var i = 0; i < incompleteTasksHolder.children.length; i++){
//set the click handler to the add task function
//call the function when the user interacts with the button
addButton.onlick = addTask;
//cycle over incompleteTaskHolder ul list item
for (var i = 0; i < incompleteTasksHolder.children.length; i++){
//bind events to list item's children (taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted);
}
//cycle over completeTaskHolder ul list item
for (var i = 0; i < completedTasksHolder.children.length; i++){
//bind events to list item's children (taskIncomplete);
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete);
}
Whole code block below
//Problem: User interaction doesn't provide desired results.
//Solution: Add interactivity so the user can manage daily tasks.
var taskInput = document.getElementById("new-tasks"); //new-tasks
var addButton = document.getElementsByTagName("button")[0]; //first button
var incompleteTasksHolder = document.getElementById("incompleted-tasks"); //incompleted-tasks
var completedTasksHolder = document.getElementById("completed-tasks"); //completed-tasks
//add a new task
var addTask = function(){
console.log("Add Task...");
//when a button is pressed create a new task
//create a new list item with the text from #new-task:
//create input with a checkbox
//input (checkbox)
//label
//input (text)
//button.edit
//button.delete
//Each element has to be modified and appended
}
//edit an existing task
var editTask = function(){
console.log("Edit Task...");
//if the class of the parent is in .editMode
//switch from .editMode
//the label text becomes input's the value
//else
//switch to .editMode
//the label value becomes label's the text
//toggle .editMode on the parent
}
//delete an existing task
var deleteTask = function() {
console.log("Delete Task...");
//when the delete button is pressed
//remove the parent (li) list item from the (ul)unordered list
}
//mark a task as complete
var taskCompleted = function(){
console.log("Task Complete ...");
//when checkbox is checked
//append the task list item to the #completed-tasks
}
//mark a task as incomplete
var taskIncomplete = function(){
console.log("Task Incomplete...");
//when checkbox is unchecked
//append the task list item to the #incompleted-tasks
}
var bindTaskEvents = function(taskListItem, checkboxEventHandler){
console.log("Bind list item events");
//select taskListItem's children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind editTask to edit button
editButton.onclick = editTask;
//bind deleteTask to the delete button
deleteButton.onclick = deleteTask;
//bind checkboxEventHandler to checkbox
checkBox.onchange = checkBoxEventHandler;
}
//set the click handler to the add task function
//call the function when the user interacts with the button
addButton.onlick = addTask;
//cycle over incompleteTaskHolder ul list item
for (var i = 0; i < incompleteTasksHolder.children.length; i++){
//bind events to list item's children (taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted);
}
//cycle over completeTaskHolder ul list item
for (var i = 0; i < completedTasksHolder.children.length; i++){
//bind events to list item's children (taskIncomplete);
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete);
}
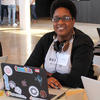
Rachél Bazelais
10,583 PointsI got it! I had the id string wong
Steven Parker
241,958 PointsSteven Parker
241,958 PointsFor code quoting, use accents/"backticks" (```), not apostrophes (
'''
).And add "js" to the top one (```js) to get syntax coloring.