Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial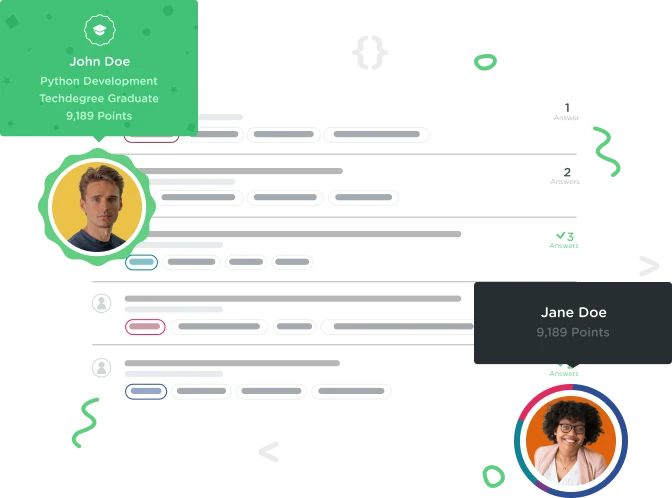
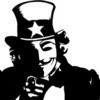
behar
10,799 PointsJava, reading from files?
So i found this simple method to read from a text document:
import java.io.*;
public class Main {
public static void main(String[] args) throws Exception {
File file = new File("pathname");
BufferedReader br = new BufferedReader(new FileReader(file));
String st;
while ((st = br.readLine()) != null)
System.out.println(st);
}
And it works great. But what if i actually wanted to modify the data in here, how would i store all of it in an array or a string?
I tried to do something like
import java.io.*;
public class Main {
public static void main(String[] args) throws Exception {
File file = new File("C:\\Users\\A\\Desktop\\test.txt");
BufferedReader br = new BufferedReader(new FileReader(file));
String test = br.readLine()
}
But now "test" will only contain the first line of the .txt file. How could i make it capture all of it? And if i do have to use the loop again, can someone please explain how it works, because i dont understand that, why does putting the vairable inside a while loop all of the sudden go thru all of it? Were not doing anything but printing inside the while loop?
1 Answer
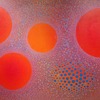
Jef Davis
29,162 PointsYou need to have your reader input line inside a while loop, similar to how it was in the first class you shared above. Each time the while evaluates as true, the console prints out the next line. As you have it written in the second class, your code is just reading the first line and stopping, so you need to check if there's more left in the file and add it to a String to save the contents of that file. Do something like:
String line;
StringBuilder file = new StringBuilder();
while ((line = br.readerline()) != null) {
file.append(line);
}
br.close();
You might also want to put the while inside of a try/catch block to handle any potential IOExceptions. Let me know if that works!
behar
10,799 Pointsbehar
10,799 PointsGot it, ty!