Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial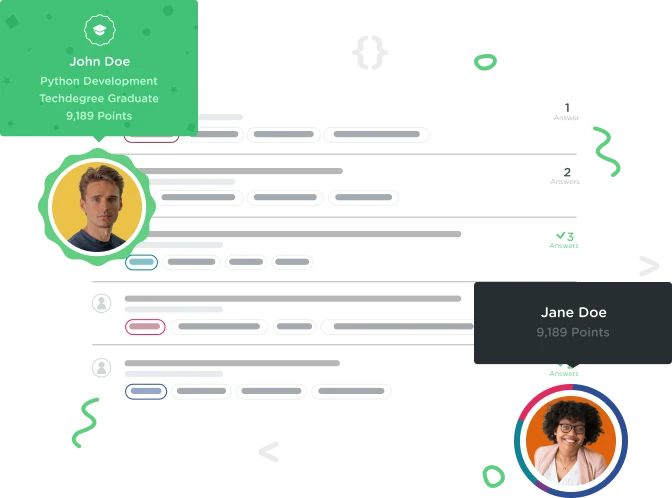

douglas gray
888 PointsI'm not sure what to put in the "else" block of my "try and except" lines of code.
the code looks like this: def add(x,y): try: float(x) + float(y) except ValueError: return none else:
I'm being asked to add an else block that returns the two added floats, but there is no variable describing this sum, retyping the sum doesn't work, and I'm not sure what else to do. Any tips?
def add(x,y):
try:
float(x) + float(y)
except ValueError:
return none
else:
return float(x) + float(y)
1 Answer
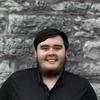
Michael Hulet
47,913 PointsThe else
block in a try
-except
-else
block runs when no exceptions were thrown in the try
block. All of the changes you made in the try
block will apply in the else
block. Take your (syntax-corrected) function, for example:
def add(x,y):
try:
x = float(x)
y = float(y)
except ValueError:
return None
else:
return x + y
If we pass in something valid, like this:
newFloat = add(5, 8)
The try
block will run and succeed in converting the numbers we passed in into float
s. All the code in the except
block will be skipped, and execution will go straight to the else
block, which adds 5.0
and 8.0
together, and returns the result. Thus, a float
value of 13.0
will be safely assigned to newFloat
.
However, let's see what happens when we pass in invalid input, like this:
invalid = add(7, "not a number")
In the try
block, Python will succeed in converting the number 7 into a float
, but it will fail when trying to convert the string "not a number"
, and it will instead raise a ValueError
. The except
block will detect that a ValueError
was encountered, and it will execute the code inside its block. Thus, the value None
will be return
ed, and the code in the else
block will never run
douglas gray
888 Pointsdouglas gray
888 PointsThank you for your thorough answer.