Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial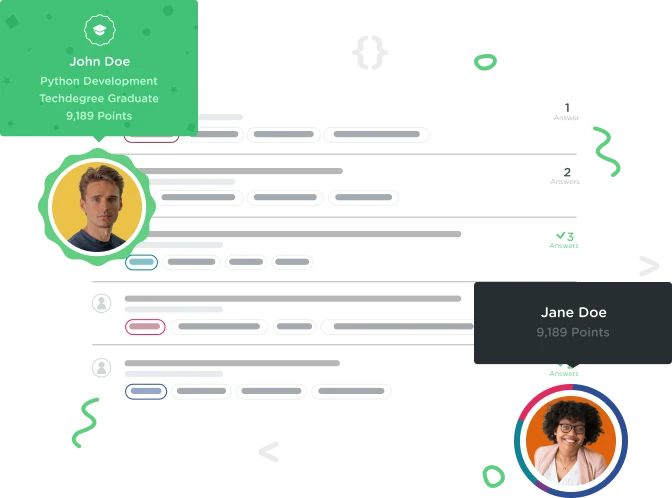

Jacob Asher
1,174 PointsI need help with this code challenge
class Point { var x: Int var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
enum Direction { case Left case Right case Up case Down }
class Machine { var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! Im a machine!")
}
} func move(_ direction: String) { switch direction { case "Up": location.y += 1 case "Down": location.y -= 1 case "Right": location.x += 1 case "Left": location.x -= 1 default: break } }
class Point {
var x: Int
var y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(_ direction: String) {
print("Do nothing! I'm a machine!")
}
}
// Enter your code below
func move(_ direction: String) {
switch direction {
case "Up":
location.y += 1
case "Down":
location.y -= 1
case "Right":
location.x += 1
case "Left":
location.x -= 1
default:
break
}
}
2 Answers
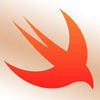
Jeff McDivitt
23,970 PointsClose but you don't need the initializer also the right is increasing by 1 and the left is decreasing by one
class Point {
var x: Int
var y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(_ direction: String) {
print("Do nothing! I'm a machine!")
}
}
// Enter your code below
class Robot: Machine {
override func move(_ direction: String) {
switch direction {
case "Up":
self.location.y += 1
case "Down":
self.location.y -= 1
case "Right":
self.location.x += 1
case "Left":
self.location.x -= 1
default:
break
}
}
}
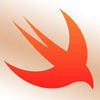
Jeff McDivitt
23,970 PointsYou need to create the class Robot that inherits from machine, you also need to override the function using the override keyword

Jacob Asher
1,174 Pointslike this? class Robot : Machine { init(x: Int, y: Int) { }
override func move(_ direction: String) {
switch direction {
case "Up":
self.location.y += 1
case "Down":
self.location.y -= 1
case "Left":
self.location.x += 1
case "Right":
self.location.x -= 1
default:
break
}
}
}