Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial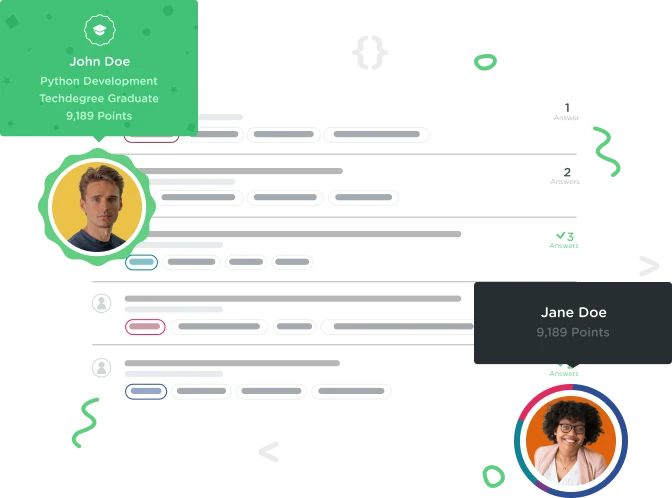

Kyle Dudley
1,970 PointsI don't understand how to handle exceptions.
So I was working on a small project to help me get the feel of Java. I was trying to handle an exception, but the issue is that in the try catch block and inside the if statement, if the condition is passed, it still throws the exception message, but my program will still run after just fine. Anyone know how to fix this?
import java.util.Scanner;
public class Calculator {
public static void main(String[] args) {
Scanner selectOperation = new Scanner(System.in);
System.out.println("Enter operation type: Addition, Subtract, Multiply, Divide");
String mathOpr = selectOperation.nextLine();
try {
if(!mathOpr.equalsIgnoreCase("Addition") ||
!mathOpr.equalsIgnoreCase("Subtract") ||
!mathOpr.equalsIgnoreCase("Multiply") ||
!mathOpr.equalsIgnoreCase("Divide")) {
throw new IllegalArgumentException("Invalid operation!");
}
} catch(IllegalArgumentException iae) {
System.out.printf("Error: %s%n", iae.getMessage());
}
if(mathOpr.equalsIgnoreCase("Addition")) {
System.out.println("Enter your first number:");
String numberOne = selectOperation.nextLine();
System.out.printf("%s added to what? %n",
numberOne);
String numberTwo = selectOperation.nextLine();
int result = Integer.parseInt(numberOne) + Integer.parseInt(numberTwo);
System.out.printf("%s added to %s equals %s%n",
numberOne, numberTwo, result);
}
if(mathOpr.equalsIgnoreCase("Subtract")) {
System.out.println("Enter your first number:");
String numberThree = selectOperation.nextLine();
System.out.printf("%s minus what?",
numberThree);
String numberFour = selectOperation.nextLine();
int resultTwo = Integer.parseInt(numberThree) - Integer.parseInt(numberFour);
System.out.printf("%s minus %s is %s%n",
numberThree, numberFour, resultTwo);
}
if(mathOpr.equalsIgnoreCase("Multiply")) {
System.out.println("Enter your first number:");
String numberFive = selectOperation.nextLine();
System.out.printf("%s times what?",
numberFive);
String numberSix = selectOperation.nextLine();
int resultThree = Integer.parseInt(numberFive) * Integer.parseInt(numberSix);
System.out.printf("%s times %s is %s%n",
numberFive, numberSix, resultThree);
}
if(mathOpr.equalsIgnoreCase("Divide")) {
System.out.println("Enter your first number:");
String numberSeven = selectOperation.nextLine();
System.out.printf("%s divided by what?",
numberSeven);
String numberEight = selectOperation.nextLine();
int resultFour = Integer.parseInt(numberSeven) / Integer.parseInt(numberEight);
System.out.printf("%s divided by %s is %s%n",
numberSeven, numberEight, resultFour);
}
}
}
2 Answers
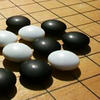
Livia Galeazzi
Java Web Development Techdegree Graduate 21,083 PointsSo there are two issues here:
-
Your if condition is wrong. You want it to be true when people type the wrong command, but instead it's true even when you type a correct command because you use || (or).
!mathOpr.equalsIgnoreCase("Addition") || !mathOpr.equalsIgnoreCase("Subtract") || !mathOpr.equalsIgnoreCase("Multiply") || !mathOpr.equalsIgnoreCase("Divide")
This is true when mathOpr is not equal to Addition OR it's not equal to Subtract OR it's not equal to Multiply OR it's not equal to Divide. It can only be false when mathOpr is equal to all four together which of course is impossible. You need to use && (and) instead.
You need to think about what should happen when the user types a wrong command How to handle an exception is just answering the question "What should happen if...?" Right now, when you throw your exception, your catch block will print a message. Then the rest of the program keeps running. I'm guessing instead you want your user to try typing a valid value again, right?
So you need the code to go back to the point where the user has to enter a value. And if the user enters a wrong value again, it should go back again. So why don't you try with a loop? You should store somewhere in a variable whether the entered value was valid or not. The loop should keep running until the value is valid. Try it.

Kyle Dudley
1,970 PointsThanks, it worked! I am going to go put this in a loop, but last time I tried and it threw the exception it made an endless loop. I did it with a do while loop, I might try a for loop.

Kyle Dudley
1,970 PointsWait never mind I get how to put in loop