Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial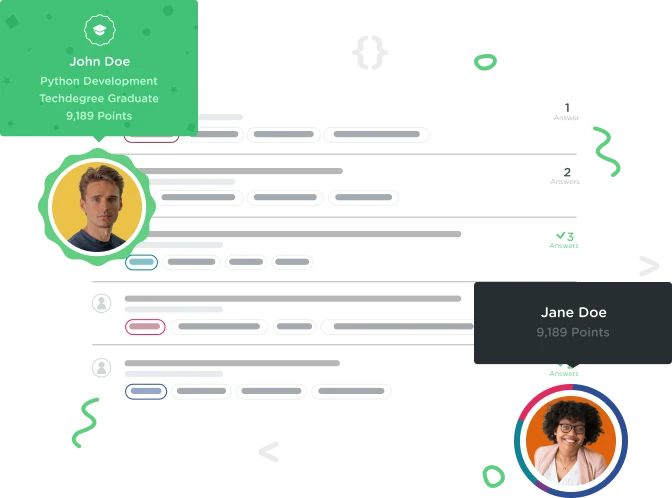
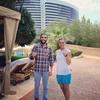
sonu dhawan
1,989 PointsHow to add an array of items into an NSMutableDictionary .
NSArray *shoeOrder = @[@"Charles Smith", @(9.5), @"loafer", @"brown",nil];
NSMutableDictionary *shoeOrderDict=[ [NSMutableDictionary alloc] init ] ;
for(NSNumber i = [shoeOrder] ; i <= [shoeOrder count];i++)
{
shoeOrderDict[@"@%",i] = [shoeOrder objectAtIndex:"%i",i];
}
1 Answer

Martin Wildfeuer
Courses Plus Student 11,071 PointsTo be honest, I did not have the time to watch the videos to this challenge, so there might be other aspects to this assignment. The straight forward way would be:
NSArray *shoeOrder = @[@"Charles Smith", @(9.5), @"loafer", @"brown"];
NSMutableDictionary *shoeOrderDict = [[NSMutableDictionary alloc] init];
[shoeOrderDict setObject:[shoeOrder objectAtIndex:0] forKey:@"customer"];
[shoeOrderDict setObject:[shoeOrder objectAtIndex:1] forKey:@"size"];
[shoeOrderDict setObject:[shoeOrder objectAtIndex:2] forKey:@"style"];
[shoeOrderDict setObject:[shoeOrder objectAtIndex:3] forKey:@"color"];
Let me walk you through your code, nevertheless:
// A: don't use NSNumber, but NSInteger, which is a primitive and
// is expected by `objectAtIndex` as well
// B: Tbh, I have no clue what you expected [shoeOrder] to return,
// but it's definitely a syntax error. My guess is you want to iterate
// over the array, but you can hardcode the 0 there, because that
// is always the first index of an array
for(NSNumber i = [shoeOrder] ; i <= [shoeOrder count]; i++) {
// [@"@%",i] is not valid syntax either, I think you wanted to do this:
// [NSString stringWithFormat:@"%i", i] to create a string from an integer
// objectAtIndex expects an NSInteger, "%i",i below is a syntax error
shoeOrderDict[@"@%",i] = [shoeOrder objectAtIndex:"%i",i];
}
That was much information, I hope it helps :)
Martin Wildfeuer
Courses Plus Student 11,071 PointsMartin Wildfeuer
Courses Plus Student 11,071 PointsOh boy, the editor totally messes up the code, so please imagine the for loop was there and written like you did it ;)
sonu dhawan
1,989 Pointssonu dhawan
1,989 PointsMuch obliged for the assistance martin.
Martin Wildfeuer
Courses Plus Student 11,071 PointsMartin Wildfeuer
Courses Plus Student 11,071 PointsSure thing :)
sonu dhawan
1,989 Pointssonu dhawan
1,989 Pointswhat about this code :
Martin Wildfeuer
Courses Plus Student 11,071 PointsMartin Wildfeuer
Courses Plus Student 11,071 PointsI recommend creating a test project in Xcode, the compiler will warn you about errors right away.