Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial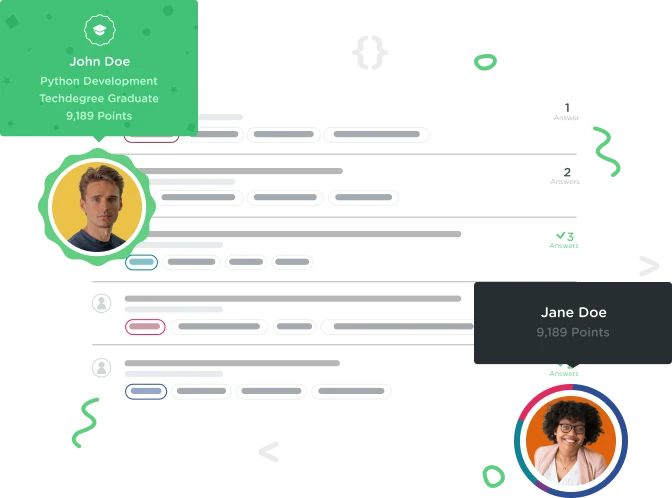

Vi Phung
2,915 PointsHi, treeHouse team, I've got an error - "Make sure you're declaring an instance method that returns a string."
// Below is my code...
struct Tag {
let name: String
}
// creating Struct named Post...
struct Post {
// adding three stored properties...
let title: String
let author: String
let tag: Tag
init(title: String, author: String, tag: Tag) {
self.title = title
self.author = author
self.tag = tag
}
// adding instance method, no param..return a String
func description() ->String {
let description = "\(title) by \(author). Filed under \(tag)"
return description
}
}
// Creating an instance of Post = let firstPost...
let someTag = Tag(name: "swift")
let firstPost = Post(title: "iOSDevelopment", author: "Apple", tag: someTag)
let postDescription = firstPost.description()
// I can't find the error.??? please help!
struct Tag {
let name: String
}
// creating Struct named Post...
struct Post {
// adding three stored properties...
let title: String
let author: String
let tag: Tag
init(title: String, author: String, tag: Tag) {
self.title = title
self.author = author
self.tag = tag
}
// adding instance method, no param..return a String
func description() ->String {
let description = "\(title) by \(author). Filed under \(tag)"
return description
}
}
// Creating an instance of Post = let firstPost...
let someTag = Tag(name: "swift")
let firstPost = Post(title: "iOSDevelopment", author: "Apple", tag: someTag)
let postDescription = firstPost.description()
4 Answers
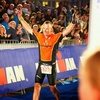
Steve Hunter
57,712 PointsThere are some silly spacing issues in there around the String
return from the description
function. I don't think that is syntactically incorrect, so I think that's just the compiler being extra picky.
This code works:
struct Post {
// adding three stored properties...
let title: String
let author: String
let tag: Tag
init(title: String, author: String, tag: Tag) {
self.title = title
self.author = author
self.tag = tag
}
// adding instance method, no param..return a String
func description() -> String { // space after the arrow, before the 'S'
return "\(title) by \(author). Filed under \(tag.name)"
}
}
let firstPost = Post(title: "iOS Development", author: "Apple", tag: Tag(name: "swift"))
let postDescription = firstPost.description()
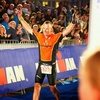
Steve Hunter
57,712 PointsHi there,
You want to output tag.name
in the string interpolation; not just the Tag
instance:
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
I also returned the string directly rather than creating a temporary constant holder for it first.
Steve.
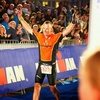
Steve Hunter
57,712 PointsYou can also create the Tag
instance inside creating the Post
instance:
let firstPost = Post(title: "iOS Development", author: "Apple", tag: Tag(name: "swift"))
let postDescription = firstPost.description()
I hope that helps,
Steve.

Vi Phung
2,915 PointsThank you Steve, that's very helpful. I didn't know you can access the member wise initialiser method using dot syntax in string interpolation.
Vi.
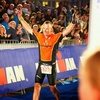
Steve Hunter
57,712 PointsHi Vi,
You're just accessing the stored property of the Tag
instance. Inside the Post
instance, there's an instance of Tag
called, imaginatively, tag
. You can access the stored properties of that instance the same way you can anywhere. So, you can interpolate tag.name
as that's just another variable/constant that can be included within the output string.
Steve.

Vi Phung
2,915 PointsI've just submitted the new codes but the error still persist. It's the same error??? I've tried the new codes in swift playground and it works perfectly. Not sure what's happening here...
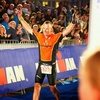
Steve Hunter
57,712 PointsPlaygrounds don't know what you are trying to pass - they just check general syntax, not the challenge output. Let me try the code ... be right back ...

Vi Phung
2,915 PointsYes, it works! Thank you for your help Steve. I've been scratching my head on this for more than a couple of hours. Now I know what coding style this console accepts. I guess I'm just too lazy to add a spaceBar there lol. Thank you again and have a good day.
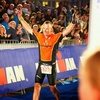
Steve Hunter
57,712 PointsGlad you got it sorted.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsYes, it is requiring a space between the arrow and the word
String
. Without it the above code fails, with it, it passes. I've added a comment in the code.