Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial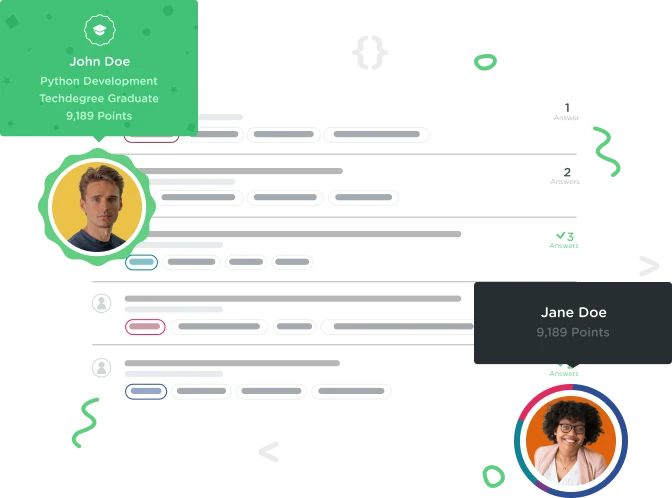
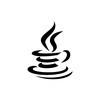
John Anselmo
Courses Plus Student 2,281 Pointserror: class, interface, or enum expected
PezDispenser.java code:
public class PezDispenser {
private final String characterName;
public static final int MAX_PEZ = 12;
private int pezCount;
public PezDispenser(String characterName) {
this.characterName = characterName;
pezCount = 0;
}
public boolean isEmpty() {
return pezCount == 0;
}
public void fill() {
fill(MAX_PEZ);
}
public void fill(int pezAmount) {
pezCount += pezAmount;
}
}
public String getCharacterName() {
return characterName;
}
public boolean dispense() {
boolean wasDispensed = false;
if(!isEmpty()){
pezCount--;
wasDispensed = true;
}
else {
System.out.println("You're out of PEZ!");
System.out.println("Filling PEZ dispenser...");
fill();
wasDispensed = false;
}
return wasDispensed;
}
}
Example.java code:
public class Example {
public static void main(String[] args) {
System.out.println("\n\nWe are making a new PEZ Dispenser");
System.out.printf("There are only %d PEZ allowed in every dispenseer.%n", PezDispenser.MAX_PEZ);
PezDispenser dispenser = new PezDispenser("Yoda");
//dispenser.characterName = "Darth Vader";
System.out.printf("The dispenser is %s %n", dispenser.getCharacterName());
if(dispenser.isEmpty()) {
System.out.println("Dispenser is empty");
}
System.out.println("Filling the dispenser with PEZ...");
dispenser.fill();
if(!dispenser.isEmpty()) {
System.out.println("Dispenser is full");
}
while(dispenser.dispense()) {
System.out.println("Chomp!");
}
if(dispenser.isEmpty()) {
System.out.println("Ate all PEZ!");
}
dispenser.fill(4);
dispenser.fill(2);
while(dispenser.dispense()) {
System.out.println("Chomp!!");
}
}
}
Error code:
./PezDispenser.java:24: error: class, interface, or enum expected
public String getCharacterName() {
^
./PezDispenser.java:26: error: class, interface, or enum expected
}
^
./PezDispenser.java:28: error: class, interface, or enum expected
public boolean dispense() {
^
./PezDispenser.java:30: error: class, interface, or enum expected
if(!isEmpty()){
^
./PezDispenser.java:32: error: class, interface, or enum expected
wasDispensed = true;
^
./PezDispenser.java:33: error: class, interface, or enum expected
}
^
./PezDispenser.java:36: error: class, interface, or enum expected
System.out.println("Filling PEZ dispenser...");
^
./PezDispenser.java:37: error: class, interface, or enum expected
fill();
^
./PezDispenser.java:38: error: class, interface, or enum expected
wasDispensed = false;
^
./PezDispenser.java:39: error: class, interface, or enum expected
}
^
./PezDispenser.java:41: error: class, interface, or enum expected
}
^
Example.java:8: error: cannot find symbol
System.out.printf("The dispenser is %s %n", dispenser.getCharacterName());
^
symbol: method getCharacterName()
location: variable dispenser of type PezDispenser
Example.java:21: error: cannot find symbol
while(dispenser.dispense()) {
^
symbol: method dispense()
location: variable dispenser of type PezDispenser
Example.java:31: error: cannot find symbol
while(dispenser.dispense()) {
^
symbol: method dispense()
location: variable dispenser of type PezDispenser
14 errors
I've scanned everything for typos, forgotten semicolons etc. Maybe I'm just blind? I'm not sure what's wrong.
2 Answers
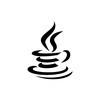
John Anselmo
Courses Plus Student 2,281 PointsNow I'm not completely sure what was wrong... but I removed both fill methods and then restarted the video and followed along again and now it's perfectly fine...

Sho Desh
948 Pointsyou must have added some extra curly braces at the end of the code. Go up to the end of the code and see if there are any xtra braces. hope it works.