Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial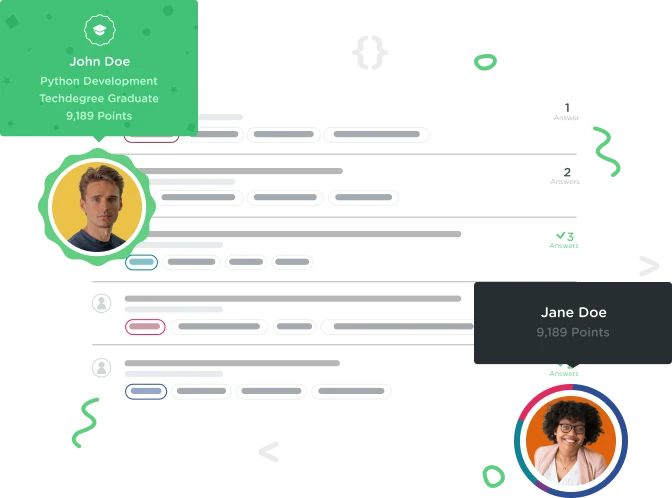

Todoroki Shoto
301 PointsDigit Ordering in Java
I was going through some random questions and encountered one that completely stumped me. Can anyone please suggest an efficient solution for the following,
Write a function to accept an integer and return a number made up of digits from the input number in ascending order. If the input is a negative, then change the order to descending.
Todoroki Shoto
301 PointsTodoroki Shoto
301 PointsThis is the code I wrote and it works but I want to make use of collections or other methods to reduce the number of lines.