Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial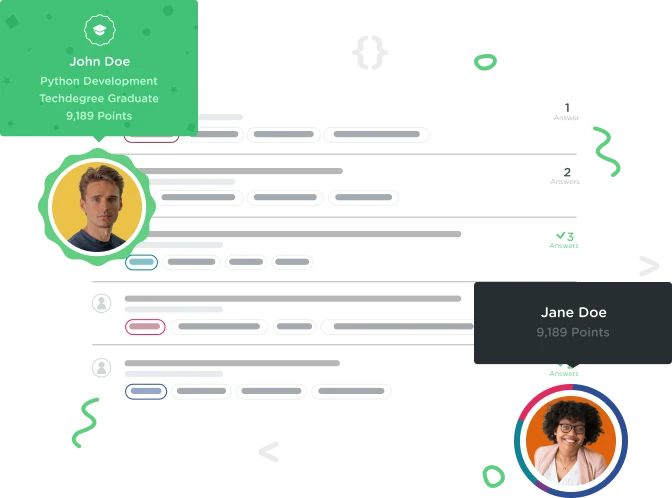
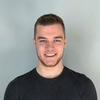
Steve Fau
5,622 PointsDeclaring the callback separately doesn't work.
The beginning of the code for reference
const astrosUrl = 'http://api.open-notify.org/astros.json';
const wikiUrl = 'https://en.wikipedia.org/api/rest_v1/page/summary/';
const peopleList = document.getElementById('people');
const btn = document.querySelector('button');
// Make an AJAX request
function getJSON(url, callback) {
const xhr = new XMLHttpRequest();
xhr.open('GET', url);
xhr.onload = () => {
if(xhr.status === 200) {
let data = JSON.parse(xhr.responseText);
return callback(data)
}
};
xhr.send();
}
// Generate the markup for each profile
function generateHTML(data) {
const section = document.createElement('section');
peopleList.appendChild(section);
section.innerHTML = `
<img src=${data.thumbnail.source}>
<h2>${data.title}</h2>
<p>${data.description}</p>
<p>${data.extract}</p>
`;
}
What is the difference between
btn.addEventListener('click', (event) => {
getJSON(astrosUrl, (json) => {
json.people.map( person => getJSON(wikiUrl + person.name, generateHTML))
})
event.target.remove()
})
and
function createHTMLelements(json) {
json.people.map( person => getJSON(wikiUrl + person.name), generateHTML)
}
btn.addEventListener('click', (event) => {
getJSON(astrosUrl, createHTMLelements)
event.target.remove()
})
The first one generates the HTML as expected. The other throws a
Uncaught TypeError: callback is not a function
which is referring to the
return callback(data)
in the getJSON() function
1 Answer

Simon Coates
8,377 PointsYour brackets:
json.people.map( person => getJSON(wikiUrl + person.name, generateHTML))
and
json.people.map( person => getJSON(wikiUrl + person.name), generateHTML)
In the second one, you're calling getJSON with a URL, but not a callback.
Steve Fau
5,622 PointsSteve Fau
5,622 PointsOmg I see.. it's always a typo :D
Idk how I managed to do this, I literally copy-pasted the line of code.
Thanks Simon.