Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial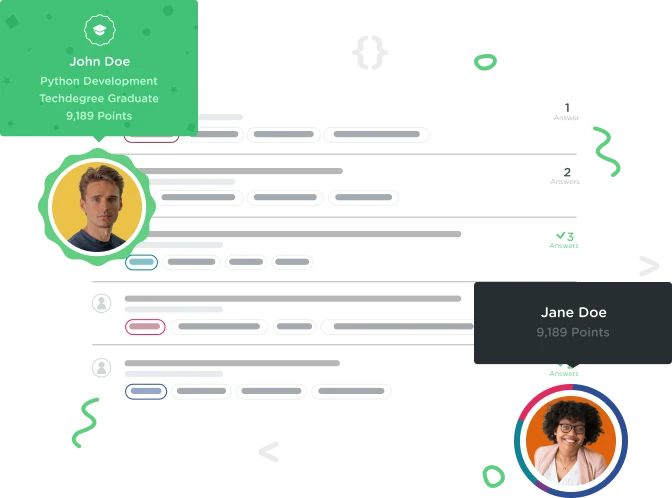

Nilo Mesa
417 PointsCan you tell me if I'm doing this right so far?
in my java 120 class my teacher wants us to write a program here it is Assignment Using Methods
Design and write a Java program that calculates and displays the results of a sales transaction. Two classes are to be defined as follows:
Class Name: Sales
Input Variables: quantity – an integer value price – a floating point value commissionRate – a double value (example: 8.5%) (to be entered as percent discountPerCent – a double value (example: 6.5%) values)
Processing: There are four input values as defined above. These variables are to be declared inside main().The main( ) method of this class should accept the quantity, price, commission rate, and discount percent from the keyboard (echo the inputs back to the screen) and call the appropriate functions described below to calculate the final sales transaction amount. main() should also display the initial sales amount.
Methods: saleAmt() – The quantity and price are passed to this method. It returns the sale amount to the calling method.
netSale() – The sale amount, commission amount, and discount amount are passed to this method. The commission amount should be added to the sale amount and the discount amount should be subtracted. Return the net sale back to the calling method.
finalSale() – This method displays the net sale amount, tax and final sales amount. **You determine appropriate argument passing and return value.
this is what I've written so far Idk if I'm doing it right.
import java.util.Scanner;
public class Sales{
public static void main(String[] args)
{
int quantity;
float price;
double commissionRate;
double discountPerCent;
Scanner Input = new Scanner(System.in);
System.out.print(" Please enter quantity ");
quantity = Input.nextInt();
System.out.print(" enter price ");
price = Input.nextFloat();
System.out.print(" enter commission rate ");
commissionRate = Input.nextDouble();
System.out.print(" enter discount percent ");
discountPerCent = Input.nextDouble();
saleAmt(quantity, price);
netSale(10, 20, 20);
}
public static double saleAmt(double qntity, double pr)
{
double saleAmount;
saleAmount = qntity * pr;
return saleAmount;
}
public static double netSale(double saleAmount, double commissionAmount, double discountAmount)
{
double AddsalAmount = saleAmount + commissionAmount;
double subComAmount = saleAmount - discountAmount;
return AddsalAmount;
}
}```
1 Answer

Yanuar Prakoso
15,196 PointsHi Nilo...
Just a couple of suggestion okay... Since this program is not finished yet.... but from what I can understand the whole process should be automated right? You need to take input from user and then calculate them using your program and then gives outputs.
However, the biggest flaw of that logic is in here:
saleAmt(quantity, price);
netSale(10, 20, 20);
This code suggest you manually set the arguments passed to the netSale(SaleAmount=10, Commision=20, discount=10. Why you even bother asking the user about commissions and discounts?
The most logical way of thinking (at least for me that is) is that your teacher wants you to calculate the SaleAmt and passed the result as argument for netSale() method along side with commisonRate and discountPercentage.
I hope this can help a little