Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial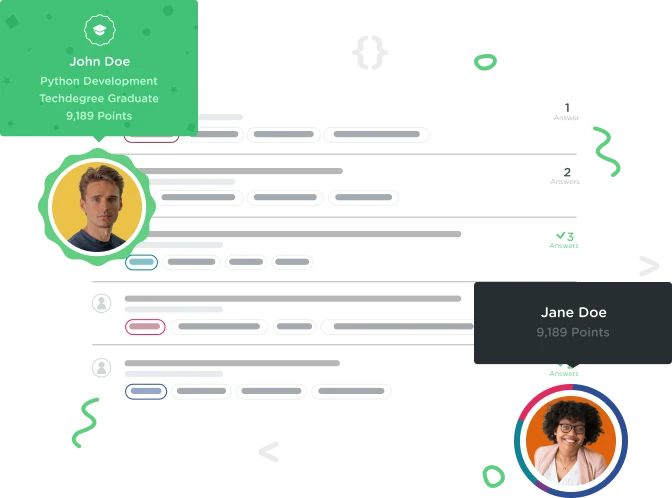

Manh Truong Nguyen
2,021 PointsCan i use lambda expression in this case
so i wrote this code for a hotel app, basically it just asked which room the customer wants to pick.
String roomchoice = scan.nextLine();
for (Room newRoom : listofRoom()) {
if (roomchoice.equals(newRoom.getNumber())){
System.out.println("Done");
break;
} else {
System.out.println("plz view list of rooms and book again");
break;
}
}
I wonder that if i can use lambda expression to make it cleaner. Something like this roomchoice.equals((Room newroom) -> ......)
2 Answers
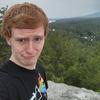
Brendon Butler
4,254 PointsSorry, I didn't even think of that as a possibility, I had assumed that getRoomList() returned a List of strings. I totally missed the "getNumber()" method you had in your original post. In my experience, lambdas are harder to read and sometimes take up just as much room as not having one at all.
String roomChoice = scan.nextLine();
getRoomList().forEach(curRoom -> {
if (roomChoice.equals(curRoom)) {
System.out.println("Done");
break;
}
});
String roomChoice = scan.nextLine();
for (Room room : getRoomList()) {
if (roomChoice.equals(curRoom)) {
System.out.println("Done");
break;
}
}
As you can see, it takes up the same amount of lines, but it's harder to understand the lambda (well not in this example, but they can get complicated and confusing). I'm not very fluent in lambdas, so there may be a better way of writing this, but this is what I came up with.
And based on the way the code is written. I would say you should have a way to check whether or not the room the user requested is a valid number. You don't need this, since the loop will run and not find a room, but it would be nice to have.
You should also have a way of checking if no rooms were found (your example will always stop on the first room). If anything, you should have more code than what you have:
boolean booked = false;
String roomChoice = scan.nextLine();
if (roomChoice.matches("^[+-]?\\d+$")) {
for (Room room : getRoomList()) {
if (roomChoice.equals(curRoom)) {
booked = true;
System.out.println("Done");
break;
}
}
}
if (!booked) System.out.println("Please view the list of rooms and try booking again");
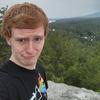
Brendon Butler
4,254 PointsThere are actually some issues with your code I will address firstly, as they cause issues or conflict with conventions.
String roomchoice = scan.nextLine(); // 1): roomchoice should be camelCased -> roomChoice
for (Room newRoom : listofRoom()) { // 3): there is an easier way to find whether or not an item is in a list. (3.1): listofRoom method is not proper grammar/conventions. It looks as if you wanted a getter so the name should either be getRoomList() or listRooms(). (3.2): "newRoom" indicates that there is a new room being created, which is not the case, this variable should be named "room" "currentRoom" "curRoom" or similar.
if (roomchoice.equals(newRoom.getNumber())){
System.out.println("Done");
break; // 2): (see below)
} else {
System.out.println("plz view list of rooms and book again");
break; // 2): having this break in your if and else statement will cause your for loop to stop after the first entry in listofRoom()
}
}
I'm not trying to attack your code or code style, just trying to offer some guidance and tips. If I were to have written this code, I would've done it this way:
String roomChoice = scan.nextLine();
if (getRoomList().contains(roomChoice)) System.out.println("Done");
else System.out.println("Please view the list of rooms and booking again");
Essentially, what I have done here is:
- CamelCased the "roomChoice" variable
- Removed your for loop as it was uneccesary
- Changed the method "listofRoom()" to "getRoomList()"
- Used a simplified if/else statement
- Reworded the responses
If you wanted to use the least lines possible, you could use a ternary operator (untested, you may need to have the user input on its own line:
// FORMAT: (if ? then : else;)
getRoomList().contains(scan.nextLine()) ? System.out.println("Done") : System.out.println("Please view the list of rooms and try booking again");
// if you do need the user input on its own line:
String roomChoice = scan.nextLine();
getRoomList().contains(roomChoice) ? System.out.println("Done") : System.out.println("Please view the list of rooms and try booking again");

Manh Truong Nguyen
2,021 PointsHi Brendon, Thanks for the commend , sorry about messy code and inconvenient name and i didn't show all my codes. So i created a class named Room and listofRoom is an arraylist of Room, that why when i tried to put your code in, it doesn't work because roomChoice is a string and it wont' work out with getRoomList().contains(roomChoice) since getRoomList is an arraylist of Room and Room has some private fields.
Brendon Butler
4,254 PointsBrendon Butler
4,254 PointsAnd just as a note, having less code is not always better. Sometimes it's worth having a bit more code, especially if it makes it more readable. For example, I used a matcher to check whether or not "roomChoice" was a number. There are better ways of doing this since this is about 10x slower than other methods and harder to read. In a simple program like this, you're not saving a lot of time by using more code, so it works here.